Semantic modules all provide the ability to log performance traces to the console, allowing you to see which aspects of the module are more or less performant and to track total init time onDomReady
All official javascript modules in Semantic are designed using a singular design pattern. This pattern allows several useful features common to all javascript components
Unlike other component libraries that hide explanations of behavior in inline comments which can only be read by combing the source, semantic modules provide run-time debug output to the JavaScript console telling you what each component is doing as it is doing it.
Semantic provides methods to set default settings, set settings at initialization, and set settings after initialization, allowing complete flexibility over component behaviors.
All events and metadata are namespaced and can be removed after initialization, modules automatically handle destroy/init events to allow users to lazy-initialize a plugin multiple times with no issues.
Modules store their instance in metadata meaning that, in a pinch, you can directly modify the instance of a UI element by modifying its properties.
Overview
Semantic does not automatically attach any events on page load. You must decide which modules to initialize on each page by initializing a module in javascript.
Example of Initializing
The following example shows how to attach behavior to elements on a page using Semantic
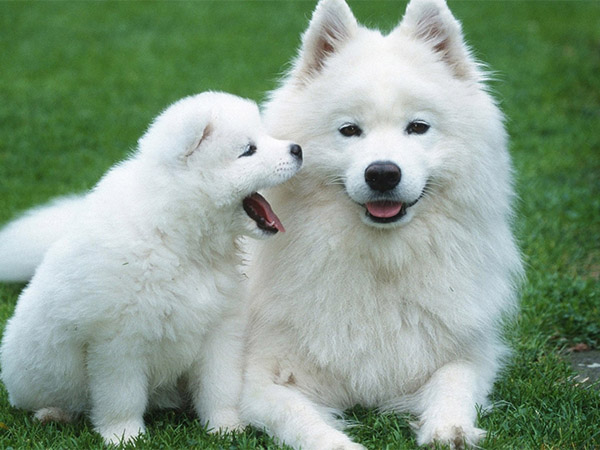
Demo Element
Using Module Behaviors
Behaviors are an element's API. They invoke functionality or return aspects of the current state for an element
Behaviors can be called using spaced words, camelcase or dot notation. The preferred method however is spaced words. Method lookup is done automatically internally.
Behaviors can be called using the syntax:
Common Behaviors
All modules have a set of core behaviors which allow you to configure the component
Name | Usage |
---|---|
initialize | Initializes an element, adding page event handlers and init data |
destroy | Removes all changes to the page made by initialization |
refresh | Refreshes cached values for a component |
setting(setting, value) | Allows you to modify or read a component setting |
invoke(query, passedArguments, instance) | Internal method used for translating sentence case method into an internal method |
debug(comment, values) | Displays a log if a user has logging enabled |
verbose(comment, values) | Displays a log if a user has verbose logging enabled |
error(name) | Displays a name error message from the component's settings |
performance log(comment, value) | Adds a performance trace for an element |
performance display | Displays current element performance trace |
Examples
Overriding Internals
Internal methods can be overwritten at run-time for individual instances of a module
Triggering Behavior
Some behaviors can accept arguments, for example a popup show behavior can accept a callback function. This arbitrary example shows opening a popup then changing its position programatically
Returning values
Behaviors may also provide an API for accessing a module's internal state. For example popups have a method is visible
which returns true or false for whether a popup is currently visible.
Unlike many javascript components, anything arbitrary in Semantic is a setting. This means no need to dig inside the internals of the component to alter an expected css selector or class name, simply alter the settings object
Common Settings
The following is a list of common settings usually found in javascript modules.
Name | Usage | |
---|---|---|
name | Name used in debug logs to differentiate this widget from other debug statements. | |
debug | Whether to provide standard debug output to console. | |
performance | Whether to provide performance logging to console of internal method calls. | |
verbose | Whether to provide extra debug output to console | |
namespace | Namespace used for DOM event and metadata namespacing. Allows module's destroy method to not affect other modules. | |
metadata | An object containing any metadata attributes used. | |
selectors | An object containing all selectors used in the module, these are usually children of the module. | |
classNames | An object containing all class names used in the module. | |
errors | A javascript array of error statements used in the plugin. These may sometimes appear to the user, but most often appear in debug statements. |
Changing Settings
The following examples use popup as an example for how to modify settings
Reading Settings
Settings can also be read programmatically: